This is part 9 of the JavaScript blog post series that will take you from beginner to advanced. This time we’ll explore the following topics: JavaScript methods, default parameter values, the Date object in JavaScript and the arrow function. By the end of this series, you will know all the basics you need to know to start coding in JavaScript. If you haven’t read the previous blog post about JavaScript objects you can do it here. Without further ado, let’s get started with the ninth tutorial.
JavaScript methods and more – table of contents:
Until now we have seen many concepts and topics in JavaScript, but there are still some commonly used ones that we did not discover. In this tutorial, we will see what they are all about. The first one is the default parameter values in JavaScript.
Default parameter values
Functions are used extremely commonly in programming, and when something is used this often there are not only frameworks like React that leverage JavaScript functions, but there are even further optimizations developed to even get more out of JavaScript functions. One of the major features we have in functions is called default parameter values. Default parameters allow us to write safer codes that can make safe presumptions about the user input. This also benefits the user by providing them a default setting that can make it easier to choose from their options as well. Let’s see some examples to that.
// assume you are developing a website for a coffee shop // and most people want to get a medium size americano // knowing this you want to make it easir for people to // order their coffee with less hustle function orderCoffee(typeOfCoffee="americano", sizeOfCoffee="medium", numberOfCoffee=1){ return (numberOfCoffee + " " + sizeOfCoffee + " size " + typeOfCoffee + " is being prepared. "); } console.log("Default order:"); console.log(orderCoffee()); // this way, when an average customer orders online, // it will be much easier for them to order their daily coffee // so much so that they will be able to order it with a single button // alternatively people can also customize their coffee // by changing the inputs console.log("Custom order:"); console.log(orderCoffee("latte", "large", 2)); console.log("Another custom order:"); console.log(orderCoffee("macchiato", "small", 1)); // it is also possible to change only part of the inputs // and leverage the default parameters // for the rest of the input fields console.log("Partially customized order:"); console.log(orderCoffee("iced coffee"));
Running the code above give us the following output:
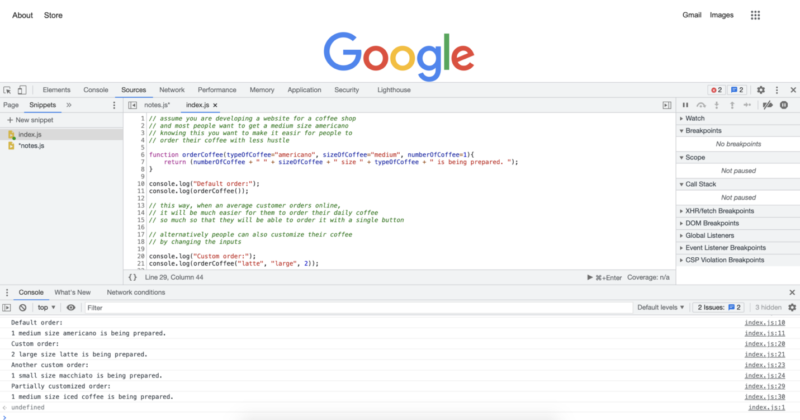
The Date object in JavaScript
The Date object in JavaScript is pretty commonly used, especially in web development. We can use the Date object to perform time-sensitive functions such as changing the display settings to dark mode, light mode or any other mode the user might prefer. We can also use the date information as needed inside the project we are working on. Here are some examples of the Date object in action:
// the first step is to create a date object instance // we cna do this by writing the following and // setting it to a variable or constant of our choice let today = new Date(); console.log("We are at year: " + today.getFullYear()); console.log("We can also get month in number:"); console.log(today.getMonth()); console.log("Also we can get the hour of the day like this:"); console.log(today.getHours()); console.log("We can also get the exact minutes along with the seconds"); console.log(today.getMinutes()); console.log(today.getSeconds()); // once we have these numbers we can use them as we like // if we want we can display them or make decisions based on them. // if we want to display month with a name // rather than a number, we can also achieve that // with the following const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; console.log("We are at the month of " + months[today.getMonth()]); // what we just did was to create an array to store month names // and then choose the correct month using an index value // provided by the .getMonth() method. // if we wanted to turn on dark mode after 8 pm, // we could do that with the following code // one of the first thing we should remember is that // hours are given in the 24 hour format // that means 8pm will mean 20 as hours // we can also use a short hand way // and combine the new date object creation // with the get hours method let timeOfDay = new Date().getHours(); if (timeOfDay >= 20) { console.log("Turning on Dark Mode..."); } else { console.log("Do not turn on the Dark Mode"); } // since current time is over 8pm, // in this case we expect to get turn on Dark Mode. // which is also the reuslt we get as we can see from // the console output.
Running the code above will give us the following console logs:
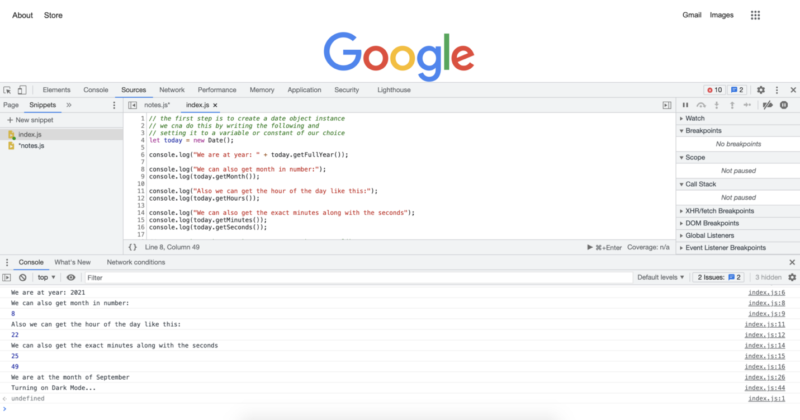
Map() method
The map method is a highly useful method that can save you many lines of code, and depending on how you use it can make your code much cleaner. It essentially replaces using a for loop when you use it to loop over an array. Here are some examples to map() method.
// lets create an array we will use for mapping let someNumbers = [1, 2, 3, 4, 5]; // lets also create the functions we will // provide to the map method function doubleNums(num1){ return num1 * 2; } function squareNums(num1){ return num1 * num1; } function add100(num1){ return num1 + 100; } console.log("Doubled numbers array:"); console.log(someNumbers.map(doubleNums)); console.log("Squared numbers array:"); console.log(someNumbers.map(squareNums)); console.log("100 added to each of the element in the umbers array:"); console.log(someNumbers.map(add100)); // map() method will loop over each of the // items in a given array and apply the // provided function // note that we do not include paranthesis // after the function names, this would call the function // instead we pass the function name, // and map() method calls them when it needs to
Running the code above will give us the following console logs:
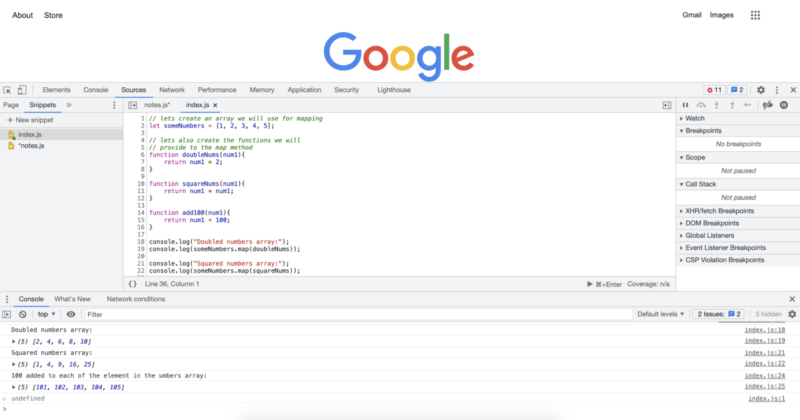
Filter() Method
The filter() method, together with the map() method are both pretty common JavaScript methods. They are very similar to the map() method we have just seen. With the map() method we can pass in any function, and that function is being applied to each of the elements in an array. With the filter() method, we will pass in an filter criteria and the filter method will loop over all the items in an array and it will return a new array with only the items that pass the criteria will remain. Let’s see some examples to that:
// lets first create an array // to apply the filter() method let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; function checkEven(num1){ if (num1 % 2 == 0){ return num1; } } function checkOdd(num1){ if (num1 % 2 == 1){ return num1; } } function over13(num1){ if (num1 > 13){ return num1; } } function divisibleByFive(num){ if (num % 5 == 0){ return num; } } console.log("Even numbers from the list:"); console.log(someNumbers.filter(checkEven)); console.log("Odd numbers from the list:"); console.log(someNumbers.filter(checkOdd)); console.log("Numbers over 13 from the array:"); console.log(someNumbers.filter(over13)); console.log("Numbers divisible by 5 from the array:"); console.log(someNumbers.filter(divisibleByFive));Running the code above will give us the following console logs:
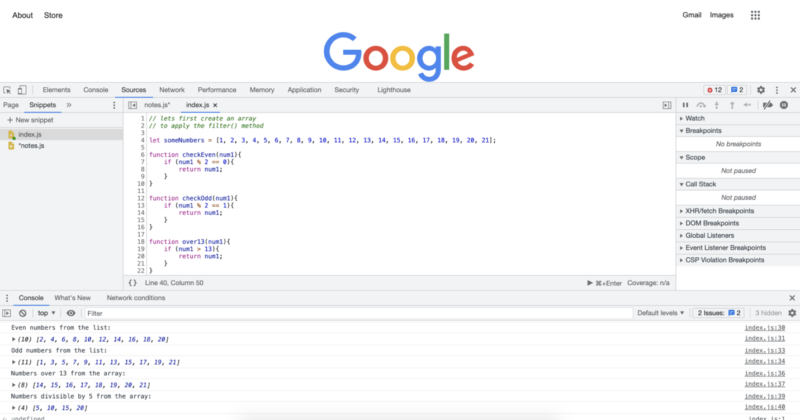
Arrow function
Remember when we said that functions are extremely common in JavaScript and there are many optimizations are made to them to even get more performant or clean code? Well, arrow functions are one of them. Arrow functions are sometimes also referred to as the fat arrow. They essentially provide a much shorter way to write your functions. They are also very commonly used with the JavaScript methods that we have just seen. Let’s see them with some examples:
// JavaScript provides multiple levels of // code shortening with arrow functions depending on your exact code // Essantially the longest way we can write a function is // the way we always write them without using the arrow functions // lets start with an array to apply the arrow functions let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; console.log("Original Array:"); console.log(someNumbers); // in the previous examples we have applied many functions // after creating them as regular named functions // In this example we will apply the exact transformations // so that you can see the both extremes in code lenght // double every number in the array: console.log("Double every number in the array:") console.log(someNumbers.map(num => num * 2)); // square every number in the array: console.log("Square every number in the array:") console.log(someNumbers.map(num => num * num)); // add 100 to every number in the array: console.log("Add 100 to every number in the array:") console.log(someNumbers.map(num => num + 100)); // Only keep the even numbers in the array: console.log("Only keep the even numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 0)); // Only keep the odd numbers in the array: console.log("Only keep the odd numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 1)); // Only keep the numbers that are evenly divisible by 5: console.log("Only keep the numbers that are evenly divisible by 5:") console.log(someNumbers.filter(num => num % 5 == 0)); // Only keep the numbers that are over 13: console.log("Only keep the numbers that are over 13:") console.log(someNumbers.filter(num => num > 13));
Running the code above will give us the following console logs:
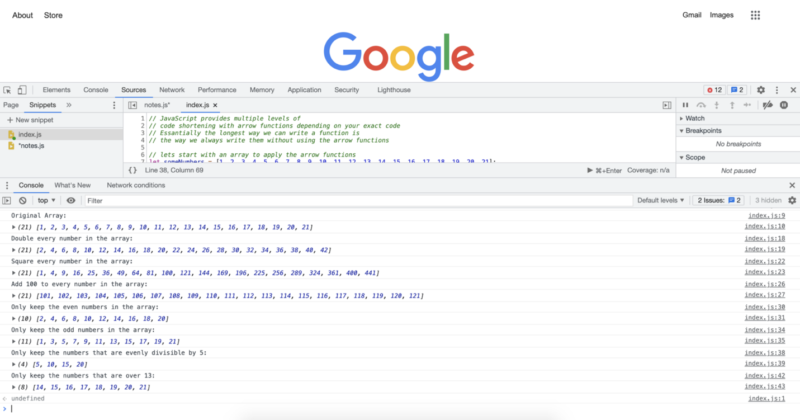
In the next tutorial we will have an overview of what we have seen and see what is next.
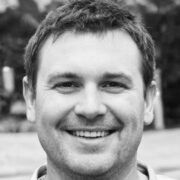
Author: Robert Whitney
JavaScript expert and instructor who coaches IT departments. His main goal is to up-level team productivity by teaching others how to effectively cooperate while coding.