This is part 3 of the JavaScript blog post series that will help you explore the topic of variables and data types in JavaScript. By the end of this series you will know all the basics you need to know to start coding in JavaScript. Without further ado, let’s get started with the third tutorial.
Variables and data types in JavaScript – table of contents:
In this blog post we will continue with where we left from the second blog post. At this stage you should have your Google Chrome browser open and more specifically have your JavaScript console open. If you for some reason closed them, it would be a good time to open them back up.
If you are on a Mac, the keyboard shortcut to open up the console is to press “Option + Command + J”, after you open up Chrome. If you are using a Windows device, you can use the keyboard shortcut of “Control + Shift + J”, to open up the JavaScript Console, once you open up Chrome. Or you can also go to the menu at the top and go to View -> Developer -> JavaScript Console.
Variables
Variables in JavaScript or any other programming language are extremely common. And it is for a good reason. Variables have a very critical role in programming. In a nutshell, variables allow you to store some data to move the data around, modify it, update or delete it in your program. It is such an important concept in fact that there are 3 ways you can create variables. The programming lingo for creating variables is called “declaring variables”, which basically means that we are declaring a variable in computers memory.
To create the variables we will use one of the 3 keywords and they are: “var”, “let” and “const”. One of the first things you should know about these 3 keywords is that until 2015, only the “var” keyword was used and the “let” and “const” keywords were introduced with a JavaScript standard called ES6 or ES2015.
If you have no idea what ES6 or ES2015 here is what you should know about it. When JavaScript first came out different companies implemented different versions of JavaScript, and there had to be a standard, for your code to run predictably and reliably in all the major browsers. So an organization called ECMA, (European Computer Manufacturing Association) came up with a set of JavaScript standards that browsers should implement. So that as long as you are following those standards when you are writing JavaScript, your code should run predictably according to those standards. This standard is called EcmaScript and they have been releasing the updated standards since 1997. And since 2015, they have been releasing these standards annually, and hence the latest released standard is called ES2021 as of writing this blog post.
But not all releases are the same, some version differences are not as large, while some introduce pretty major changes to the language. The last major changes happened with ES2015 also called the ES6 as it was the release of the version six of the ECMAScript standardization. One of the significant changes came to the variable declarations.
Before ES6, only the “var” keyword was used and it was the only way to declare variables, hence the name “var”. The var keyword came with flexibilities that could cause unwanted issues. Because it was significantly flexible in use, it was easier to make mistakes with variables if you were less careful than you should be. For example if you declared a variable called “user1”, you cannot re-declare a variable called user1 with the new “let” and “const” kywords, but you could do that with the “var” keyword. And if you forget that you already have a user1 in your program, the second time you declare the user1 with another users information, you would be overriding the actual first user’s information, which would effectively delete the first user’s information.
// this can cause confusion var user1 = "John"; var user1 = "Jack"; // if you try to do the same thing // with let or const you will get an error let user1 = "John"; let user1 = "Jack"; // likewise you will also get an error // if you try to do the same thing with the const keyword const user1 = "John"; const user1 = "Jack";
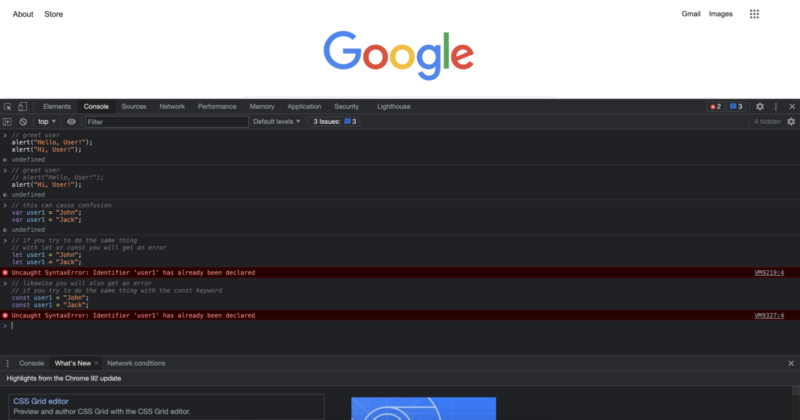
But it is not like you won’t see the “var” keyword being used anywhere, you will certainly see it especially some beginner level JavaScript courses. Especially if they are couple years old, there are a lot of tutorials that will still show you the old way of writing JavaScript. But the reality is that, it is just not the best practice to use that anymore. Instead, the best practice is to use “let” or “const” keywords when declaring variables, depending on your needs. But what needs are we talking about? In a nutshell, if you are planning to change the value inside a variable you will want to use the “let” keyword, if you know that you won’t change the value in a variable, you will want to go with “const” instead. Let’s see some examples to variable declarations using both let and const.
// we can use "let" when we want to track the game level of the user // because we know that it will change let gameLevel = 1; gameLevel = 2; gameLevel = 3; // we can use the "const" keyword when declaring user ID // because we know that we won't change it const userId = 1010101999;
If you also noticed from the above code, with the latest best practices, we only write the declaring keywords once, and we do that when we are first declaring the variable. When we want to change the value inside the variable later on, we don’t use any keyword before the variable name.
When we want to access the data that these variables or constants hold, we can simply use their name. For example, If we want to show the user their game level and their user id, we that with the following code:
// we can add the userID to the end of the sentence with a plus sign // we will explain this later on in the tutorial alert("Your user ID is: " + userId); // we can also show the user their game level like the following alert("Your current game level is: " + gameLevel); // alternatively we can directly display // the contents of the variables by showing them inside an alert alert(userId); alert(gameLevel);
Running the last two blocks of code would provide the following outputs:
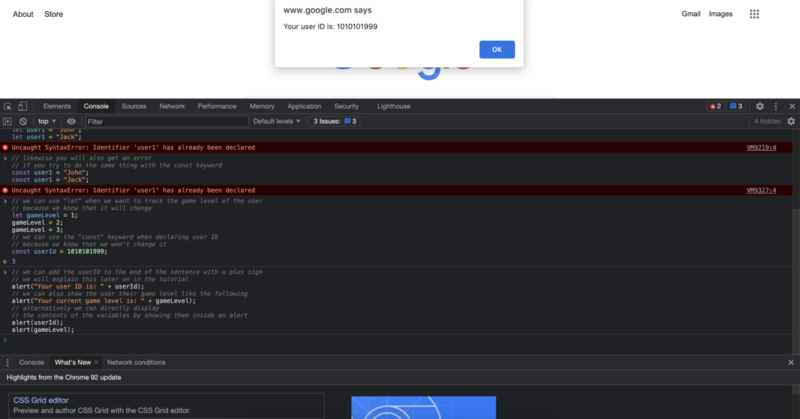
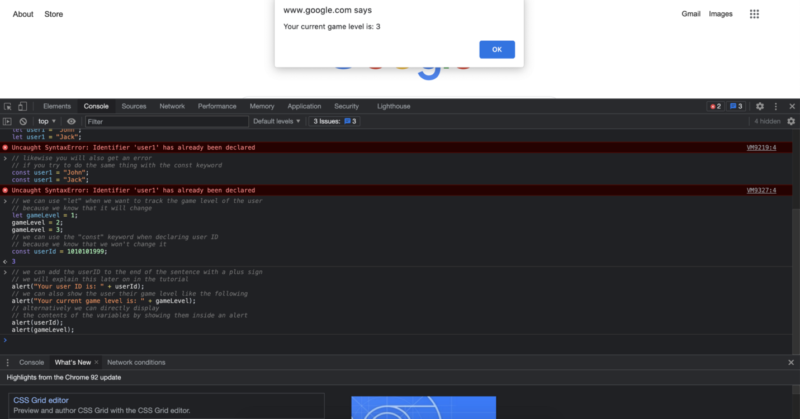
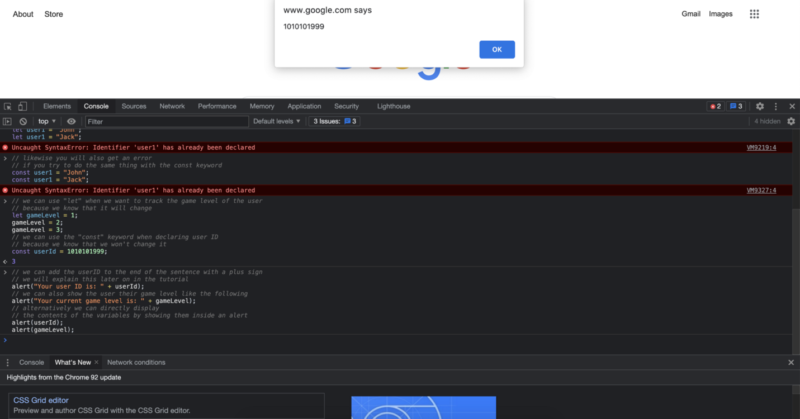
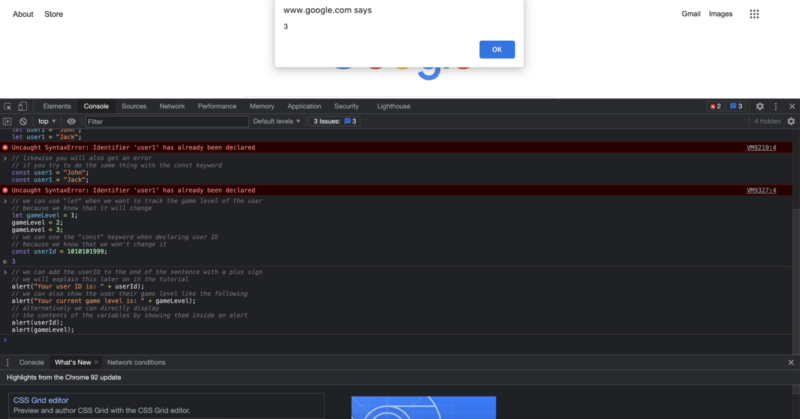
How to name your variables?
When naming your variables there are certain rules and conventions you should consider. The first consideration is that what characters can use to name your variables? Can they start or end with a number? Is there a common unspoken truth to naming your variables? Let’s answer all of that and more.
Different programming languages have different conventions for naming variables. In JavaScript the convention is to name them with what is called “camel casing”, and goes like this. If the variable name has only one word, then you just write that word in all lowercase. If there are multiple words in the variable name, then you write the first word with all lower caps and you capitalize all the subsequent words and you write them without any spaces or other signs. For example, if we are making a game, we could name the variables as such:
// a single word variable let strength = 50; // a descriptive name that includes multiple words let numberOfArrowsLeft = 145;
On top of using alphabetical characters, we can also use numbers, the dollar sign and the underscore sign in the variable names. It is important to note that you cannot start the variable name with a number, but you can end it with a number.
let some$$ = 100; let another_$ = 20; let car1 = "Tesla";
Note that just because it is possible, we don’t want to name our variables with unclear names or with symbols. And that is another topic by itself. When naming variables, the convention is to have clear and descriptive names. For example, if we are going to name a variable to denote how many arrows left in an archers bag, we should use a descriptive name such as the one we used in the example above. If we were to use just:
let x = 145;
This name would tell us nothing about the what value it holds. And even couple days after writing this code we would have to read the surrounding code to understand what that variable might mean. Thats why, both for your own clarity when writing the code, and to your future self who might review the code, it is really important that you get used to naming your variables in a clear and descriptive way. This will also become even more important when you start working with other people and show them your code.
At this point you maybe thinking that: Its great that we can move the data around and can even change it using variables. But what data are we talking about here? Why did we put parts in quotations and some parts are not in quotation? To answer all of that and more, let’s see the basic data types in JavaScript.
Basic data types in JavaScript
Different data types are good at doing different things. In this basic data types in JavaScript tutorial we will see the most basic 3 types of data which are commonly used in JavaScript. Later on in the series we will learn about other data types in JavaScript. Once you learn these first 3 basic data types, it will be much easier to learn the other data types. The 3 data types we will see in this tutorial are: Strings, Numbers and Booleans. Without further ado, let’s start with the first one.
Strings
If you have been following along with the tutorial from the beginning, you already worked with the string data type! When we wrote an alert that said “Hello, World!” that was using the string data type to store the text we wrote. In JavaScript there are 3 ways to represent strings. First one is to surround your text with the double quotation marks. Second one is to surround your text with single quotation marks. And the third one is to surround your text with back ticks. All three of them looks like this:
const string1 = "Some text here."; const string2 = 'Some text here.'; const string3 = `Some text here.`;
As you can see, the one with the “back ticks” looks pretty similar to single quotation, but it is slightly laid back. The use of back ticks to create strings is a feature that is introduced with ES6, to make it easier to work with textual data. It provides multiple advantages over the previous other two. By convention you may see the either the double quotes or the back ticks being used more often. You can find the back ticks on the left key of number 1, in your keyboard.
Use of double quotes look more familiar and it is easier to understand at the first look, but back ticks come with more advantages overall. In this example all 3 of them function the same way as it is a simple example. To display all three of them at the same time, or even in the same line, one thing we can do is to write their names and use the plus sign in between them, in a way adding the strings to each other.
alert(string1 + string2 + string3);
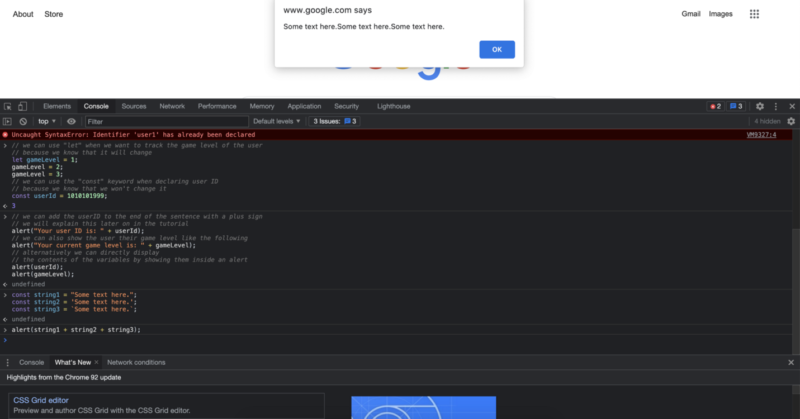
As you can see all 3 of them are displayed right after one of them ends. This is because just told the interpreter to add the strings to each other. If we want to add spaces in between them we can always add that space with another string.
alert(string1 + " " + string2 + " " + string3);
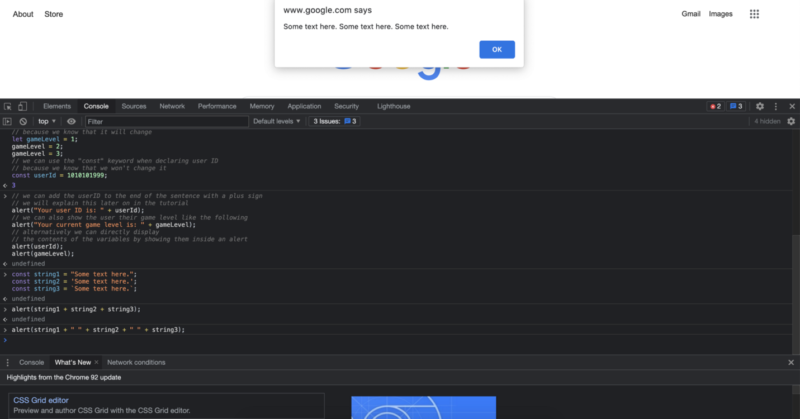
We can also check the data of a variable by writing “typeof” in front of it. For example:
alert(typeof string1);
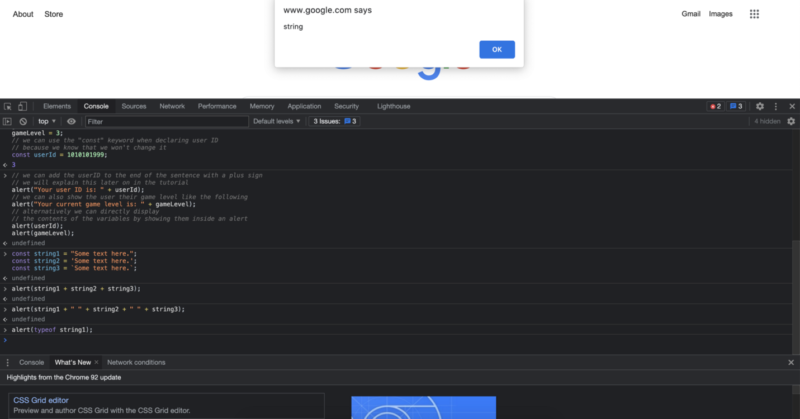
Numbers
When we are working with numbers in JavaScript we are generally using the “Number” data type. It is the most simple one to get started with and comes with almost no learning curve.
When type out a number, it is represented in the number type. And if we assign that number to a variable or a constant they will also have the data type of number.
let someNumber = 5; const score = 90; alert(typeof someNumber); alert(typeof score);
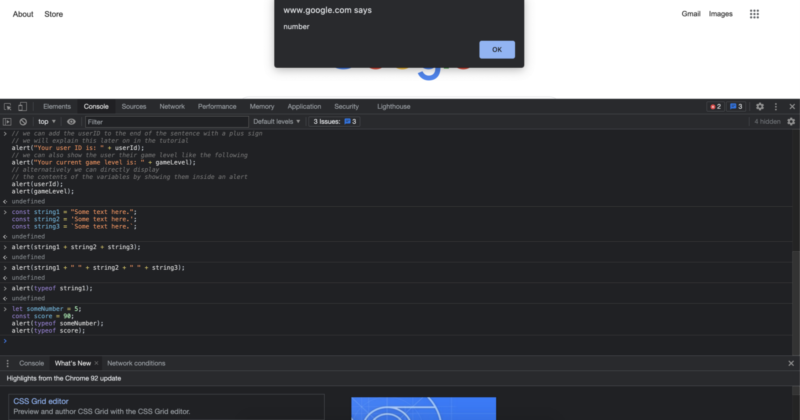
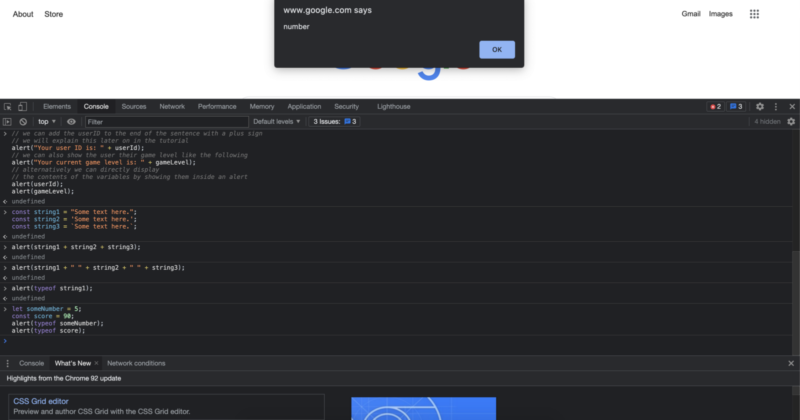
On top of regular numbers we have, JavaScript also provides other features that can help us write safer and more predictable code. For example, in JavaScript we can have something called “Infinity”, and it is exactly what it sounds like. Moreover it can be positive infinity and negative infinity. Let’s see what is looks like with an example.
// we can divide by zero and that does not throw an error // instead it returns Inifinity alert(13 / 0);
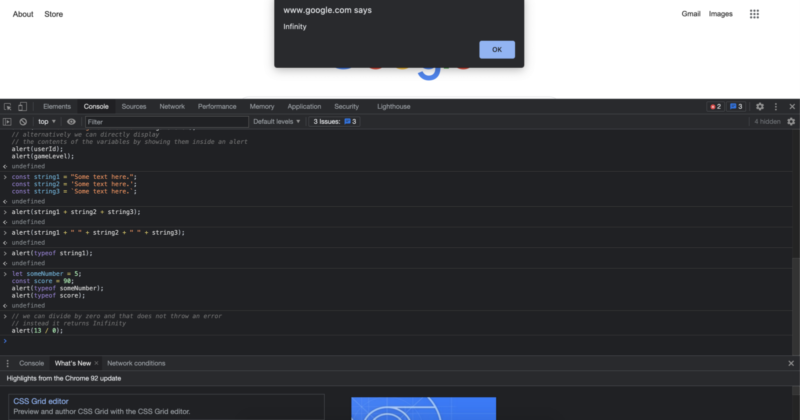
// similarly we can also get negative infinity alert(-25 / 0);
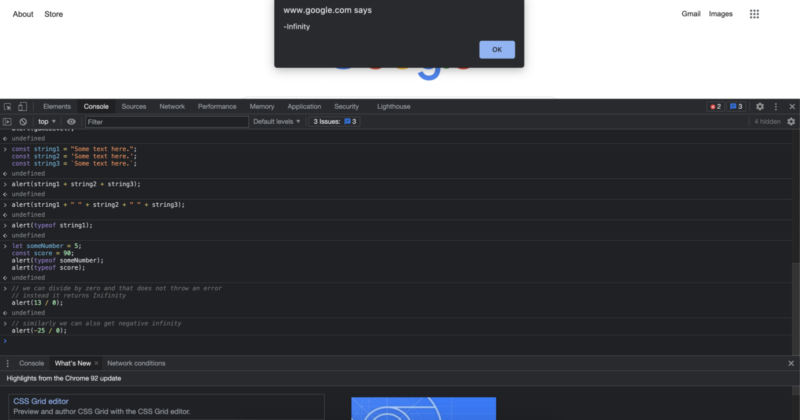
As you have already seen with couple examples, we can also do arithmetic operations with numbers. From the basic addition, subtraction, multiplication, division to even more programming specific arithmetic operations. We will learn more about them in the next tutorial.
Booleans
Booleans are extremely common in programming. Most of the time we will not explicitly use their names, but we will use their simple property under the hood. A boolean can have two values: “true” and “false” and they are exactly what they sound like. A lot of time you will find yourself writing code to make a comparison and a conclusion based on the result of that comparison.
A lot of the time these comparisons are rooted from real life scenarios, and they follow a simple logic. Is the light on or off? Is there rain outside? Are you hungry? Is this number bigger than the other number? Is this paragraph longer than the next one? Is the user on a large screen?
There are many times where you don’t just want to have a number value or string value to things, but you actually want a yes or no answer. In a nutshell, at those times, we will be using the boolean data type.
// is the first number bigger than the second one alert(13 > 12);
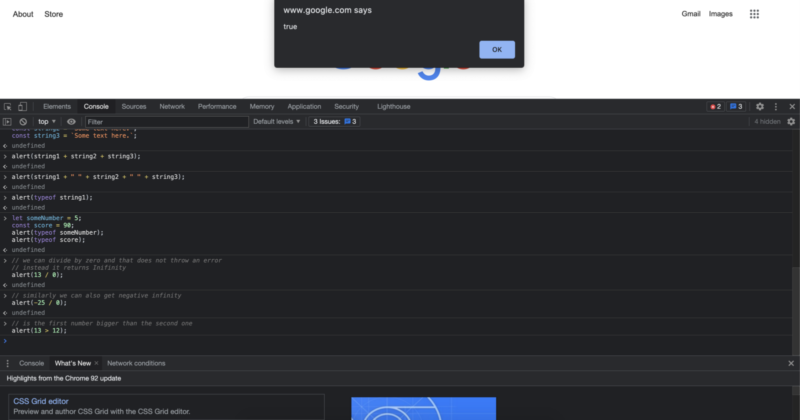
// check the variable type let isRainyOutside = true; alert(typeof isRainyOutside);
Running this code would give us the following output:
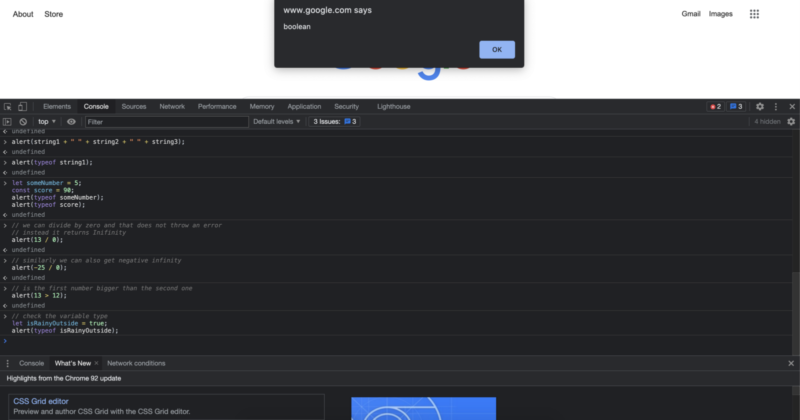
Now you know about variables and data types in JavaScript. In the next blog post we will use these data types to start making decisions in our code and so much more!
If you like our content don’t forget to join Facebook community!
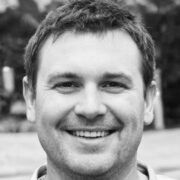
Author: Robert Whitney
JavaScript expert and instructor who coaches IT departments. His main goal is to up-level team productivity by teaching others how to effectively cooperate while coding.