This article will help the reader understand about the basic Python sets and dictionaries with some basic applications in real world. We will be using Visual Studio Code as our code editor. If you have not installed Visual Studio Code, the instructions are given in the previous blog.
Python sets and dictionaries – table of contents:
- Python sets
- Operations in Python sets
- Dictionaries in Python
- Difference Between Python sets and dictionaries
Python sets
A set is a mutable and unordered collection of unique elements. Set is written with curly brackets ({}), being the elements separated by commas.
It can also be defined with the built-in function set([iterable]). This function takes as argument an iterable (i.e., any type of sequence, collection, or iterator), returning a set containing unique items from the input (duplicated elements are removed).
For Example:
# Create a Set using # A string print(set('Dev'))
Output: {'e', 'v', 'D'}
# a list set(['Mayank', 'Vardhman', 'Mukesh', 'Mukesh'])
Output: {'Mayank', 'Mukesh', 'Vardhman'}
# A tuple set(('Lucknow', 'Kanpur', 'India'))
Output: {'India', 'Kanpur', 'Lucknow'}
# a dictionary set({'Sulphur': 16, 'Helium': 2, 'Carbon': 6, 'Oxygen': 8})
Output: {'Carbon', 'Helium', 'Oxygen', 'Sulphur'}
Now, we know how to create Sets. Let’s see what the common operations in sets are.
Operations in Python sets
Adding an element in a set
Syntax for adding element is set.add(element).
The method works in-place and modifies the set and returns ‘None’.
For Example:
locations = set(('Lucknow','kanpur','India')) locations.add('Delhi') print(locations)
Output: {'India', 'Delhi', 'Lucknow', 'kanpur'}
In Python sets, we cannot insert an element in a particular index because it is not ordered.
Removing an element from a set
There are three methods using which you can perform the removal of an element from a set.
They are given below:
- set.remove(element)
- set.descard(element)
- set.pop()
Let’s understand it by looking at an example for each implementation:
set.remove(element)locations = set(('Lucknow', 'kanpur', 'India')) #Removes Lucknow from the set locations.remove('Lucknow') print(locations)
Output: {'India', 'kanpur'}set.discard(element)
locations = set(('Lucknow', 'kanpur', 'India')) # Removes ‘Lucknow’ from the set locations.discard('Lucknow') print(locations)
Output: {'India', 'kanpur'}
As you can see that both the ‘remove’ and ‘discard’ method work in-place and modify the same set on which they are getting called. They return ‘None’.
The only difference that is there in the ‘remove’ and ‘discard’ function is that ‘remove’ function throw an exception (KeyError) is raised, if ‘element’ is not present in set. The exception is not thrown in case of ‘discard’.
set.pop()locations = set(("Lucknow", 'Kanpur', 'India')) # Removes ‘Lucknow’ from the set removed_location = locations.pop() print(locations) print(removed_location)
Output: {'Kanpur', 'Lucknow'} India
‘pop’ function does not take any arguments and removes any arbitrary element from set. It also works in-place but unlike other methods it returns the removed element.
So, we have covered lists, tuples, and Python sets. Now, finally let’s see how things work in python dictionaries.
Dictionaries in Python
Python dictionaries are a fundamental data type for data storage and retrieval.
The dictionary is a built-in data structure that stores key:value pairs and can be accessed by either the key or the value. Python dictionaries are unordered, and keys cannot be negative integers. On top of that, while keys must be immutable, values do not have to be.
The syntax for creating a dictionary is to place two square brackets after any sequence of characters followed by a colon (e.g., {‘a’: ‘b’}); if you are passing in more than one sequence then you need to put them in separate sets of brackets (e.g., {‘a’: ‘b’, ‘c’: ‘d’}).
For Example:
# Creating an empty Dictionary Dictionary = {} print("Empty Dictionary: ") print(Dictionary)
Output: Empty Dictionary: {}
We can also create a dictionary using in=built function known as ‘dict()’.
Let’s see how we can create it:
# Creating a Dictionary # With dict() method Dictionary = dict({1: 'Hello', 2: 'World', 3: '!!!'}) print("\nDictionary by using dict() method: ") print(Dictionary)
Output: Dictionary by using dict() method: 1: 'Hello', 2: 'World', 3: '!!!'}
Now, let’s create the dictionary using a list of tuples of key and value pair:
# Creating a Dictionary Dict = dict([(1, 'Hello'), (2, 'World')]) print("\nDictionary by using list of tuples of key and value as a pair: ") print(Dict)
Output: Dictionary by using list of tuples of key and value as a pair: {1: 'Hello', 2: 'World'}
Remember that the keys are case sensitive.
Let’s see briefly what are the methods that are present in dictionary of Python.
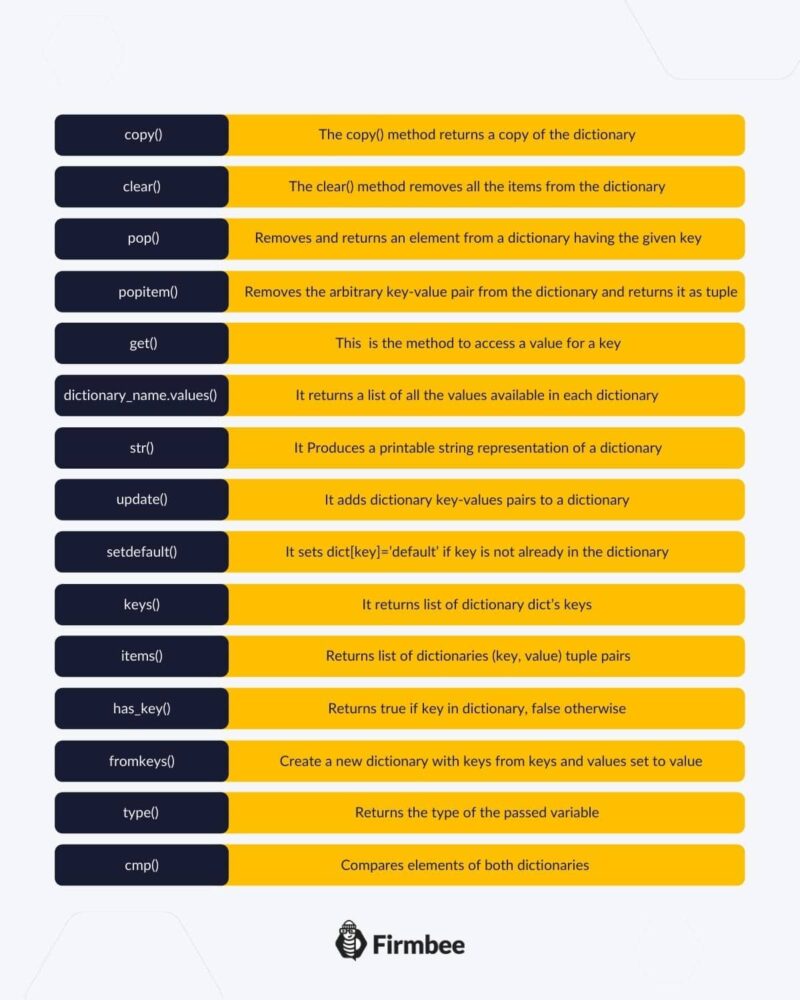
Difference Between Python sets and dictionaries
A set is a collection of values, not necessarily of the same type, whereas a dictionary stores key-value pairs.
Python sets are collections of data that do not have any order or keys. A set does not store any data about its members other than their identity. Dictionaries are collections that map unique keys to values. Furthermore, dictionaries store information about their members including the key and value pair.
So, we built some basic understanding about Lists, Tuples, Sets and Dictionaries in Python. We also investigated some functions and their implementations.
You may also like our JavaScript Course from Beginner to Advanced.
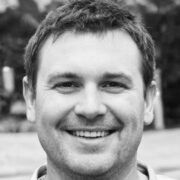
Author: Robert Whitney
JavaScript expert and instructor who coaches IT departments. His main goal is to up-level team productivity by teaching others how to effectively cooperate while coding.
Python Course From Beginner to Advanced in 11 blog posts:
- Introduction to Python Course. Part 1 Python Course from Beginner to Advanced in 11 blog posts
- Variables and Data Types in Python. Part 2 Python Course from Beginner to Advanced in 11 blog posts
- Python tuples, lists, sets and dictionaries. Part 3 Python Course from Beginner to Advanced in 11 blog posts
- Python sets and dictionaries. Part 4 Python Course from Beginner to Advanced in 11 blog posts
- Conditional statements in Python. Part 5 Python Course from Beginner to Advanced in 11 blog posts
- Loops in Python. Part 6 Python Course from Beginner to Advanced in 11 blog posts
- Python functions. Part 7 Python Course from Beginner to Advanced in 11 blog posts
- Advanced functions in Python. Part 8 Python Course from Beginner to Advanced in 11 blog posts
- Python classes and objects. Part 9 Python Course from Beginner to Advanced in 11 blog posts
- Files in Python. Part 10 Python Course from Beginner to Advanced in 11 blog posts
- Python applications in practice. Part 11 Python Course from Beginner to Advanced in 11 blog posts