This article will help the reader understand about the basic Python files and file handling along with some basic applications in real world. We will be using Visual Studio Code as our code editor. If you have not installed Visual Studio Code, the instructions are given in the first blog.
Python files – table of contents:
- Files in Python – definition:
- Examples of binary files in Python
- Examples of text files in Python
- Operations on files in Python
- Functions involved in reading files in Python
Files in Python – definition:
A file is an entity that stores information. This information may be of any type such as text, images, videos, or any music. In python, there are functions inbuilt which can be used to perform operations on files.
Examples of binary files in Python:
- Document files: .pdf, .doc, .xls etc.
- Image files: .png, .jpg, .gif, .bmp etc.
- Video files: .mp4, .3gp, .mkv, .avi etc.
- Audio files: .mp3, .wav, .mka, .aac etc.
- Database files: .mdb, .accde, .frm, .sqlite etc.
- Archive files: .zip, .rar, .iso, .7z etc.
- Executable files: .exe, .dll, .class etc.
Examples of text files in Python:
- Web standards: html, XML, CSS, JSON etc.
- Source code: c, app, js, py, java etc.
- Documents: txt, tex, RTF etc.
- Tabular data: csv, tsv etc.
- Configuration: ini, cfg, reg etc.
Operations on files in Python
Opening a file in Python:
The open() function in python is used for opening files. This function takes two arguments, one is the filename and the other one is the mode of opening. There are many modes of opening such as read mode, write mode, and others.
Let’s explore the syntax:
# File opening in python File=open(“filename”,”mode”)Modes of file opening:
“r”:– this is used for opening a file in read mode.
“w”: – this is used for opening a file in write mode.
“x”: – this is used for exclusive file creation. If the file is not present, it fails.
“a”: – this is used when you want to append a file without truncating the file. If the file is not present, then this creates a new file.
“t”: – this is used for opening file in text mode.
“b”: – this is used for opening file in binary mode.
“+”: – this is used when the user wants to update a file.
Note:
The operations for binary files are as given below.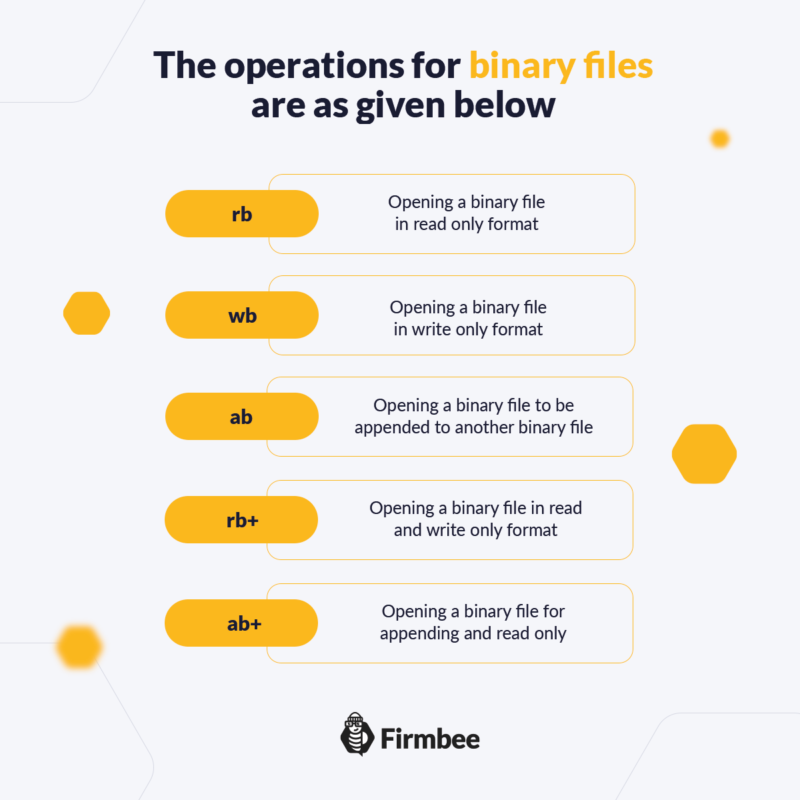
Let’s open a file using above discussed methods. The code is illustrated below. As we don’t have any file, we will create a file and then open it.
x="new file opening" with open("new","w") as f: f.write(x)
In the above code, we are creating a string variable x which contains the text “new file opening”, this string variable is being written into a file “new” using write method. We are using “with” here as it handles closing of the file. So, we are opening a file in write format and writing the string x to the file.
Now, let’s read the same file.
x="new file opening \n writing new file" with open("new","r") as f: print(f.read())
In the above code, we are opening the file new which we wrote in the previous code and opening it in read format. Note that, we are using read() function to read the file. Let’s run and see the output.
#output New file is opening
Functions involved in reading files in Python
There are three functions involved in the reading operation performed on files.
Read():This function is used when the user wants to read all the information inside the file.
x="new file opening \n writing new file" with open("new","r") as f: print(f.read())Readline():
This function is used when the user wants to read the file line by line.
x="new file opening \n writing new file" with open("new","r") as f: print(f.readline())Readlines():
This function reads all the lines but in a line by line fashion which increases its efficiency in handling memory.
x="new file opening \n writing new file" with open("new","r") as f: print(f.readlines())
Appending a file:
As discussed above, we will be opening a file in append mode which “a+” for appending it. The code is illustrated below.
x="new file opening" with open("new","a+") as f: f.write("Hello world")
Reading the file to see the appended line: x="new file opening" with open("new","r") as f: print(f.read())
Let’s explore the output:
new file openingHello world
Renaming a file:
For renaming a file, we will be using the methods present in the “os” module of python. The code is illustrated below.
import os os.rename("new.txt","example.txt")
In the above code, we are importing the “os” module and using “rename” method to rename the file we create from “new” to “example”.
Removing a file:
For removing files, we will be using the same module “os” which we have used for renaming the file. The example of the code is illustrated below.
import os os.remove("example.txt")
Copying a file:
For copying the file, we will be using the same module “os” which we have used for renaming and removing a file. The example of the code is illustrated below.
import os os.system("cp example example1")
Moving a file:
For moving the file, we will be using the same module “os” which we have used above. The example of the code is illustrated below.
import os os.system("mv source destination")
In this blog, we have covered some basics when it comes to files in Python. In the next blog post we will use all the gathered knowledge in practice.
You may also like our JavaScript Course from Beginner to Advanced.
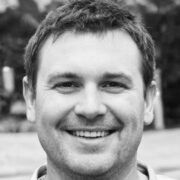
Author: Robert Whitney
JavaScript expert and instructor who coaches IT departments. His main goal is to up-level team productivity by teaching others how to effectively cooperate while coding.
Python Course From Beginner to Advanced in 11 blog posts:
- Introduction to Python Course. Part 1 Python Course from Beginner to Advanced in 11 blog posts
- Variables and Data Types in Python. Part 2 Python Course from Beginner to Advanced in 11 blog posts
- Python tuples, lists, sets and dictionaries. Part 3 Python Course from Beginner to Advanced in 11 blog posts
- Python sets and dictionaries. Part 4 Python Course from Beginner to Advanced in 11 blog posts
- Conditional statements in Python. Part 5 Python Course from Beginner to Advanced in 11 blog posts
- Loops in Python. Part 6 Python Course from Beginner to Advanced in 11 blog posts
- Python functions. Part 7 Python Course from Beginner to Advanced in 11 blog posts
- Advanced functions in Python. Part 8 Python Course from Beginner to Advanced in 11 blog posts
- Python classes and objects. Part 9 Python Course from Beginner to Advanced in 11 blog posts
- Files in Python. Part 10 Python Course from Beginner to Advanced in 11 blog posts
- Python applications in practice. Part 11 Python Course from Beginner to Advanced in 11 blog posts