We have covered the basic data types, advanced data types and conditional statements in Python in our previous blogs. In this blog, the loops will be covered. If you are new to Python, please start from the first blog to get a better understanding of this topic.
Loops in Python – table of contents:
- Loops in Python
- For loop in Python
- For loops in list
- Iterating a set using for loop
- Iterating a tuple using for loop
- Nested loops in Python
- While Loops in Python
Loops in Python
Loops are used when there is a need to do a task more than one time. For example, printing numbers from 1 to 100 or a better example would be to sum all the elements in a list or an array. Sometimes there is a need to write more than 1 loop or loop inside a loop. In Python writing these loops is very simple and even the syntax is easy to understand. As we have seen, in Python we don’t need to declare a variable first before using it. The basic looping starts with for loop. Let’s understand “for” loop.
For loop in Python
In a for loop, we have three things which needs to be mentioned. First one is the initial value of the variable on which the iteration needs to be done, the stopping condition and the last one is by how many steps you want to increment or decrement the iterator.
Let’s see the syntax of a “for” loop:
# For Loop for var in range(10): print(var) for var in range(0,10,1): print(var)
In the above code illustration, we can see that for loops are giving the same result. The syntax in the end where we provided the function range has three arguments which we discussed in the previous paragraph. In the above example the range has 0,10,1 in which 0 is the initial value of the iterator, 10 is the final value but range actually iterates till 10-1 which is 9 and 1 is the incrementing of the iterator every time the loop runs.
Let’s run the above program
Output: 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9
As we can see from the output illustration, it’s printing 0 to 9 numbers.
For loops in List
In a list we have a collection of items and below is the illustration on how to use for loops to iterate through a list.
X=[1,2,3,4,5,6] for i in X: print(i)
Output: This will print all the elements in the list. 1,2,3,4,5,6
To include the index also while printing, the code is illustrated below.
X=[1,2,3,4,5,6] for i in range(len(X)): print(i,X[i])
This will print both index and the value in the list.
There is an easy way to get the index and value using enumerate function. The enumerate function use is illustrated below.
X=[1,2,3,4,5,6] for i,value in enumerate(X): print(i,value)
Output: 0,1 1,2 2,3 3,4 4,5 5,6
Iterating a set using for loop
Iterating a set is like the list iteration using for loop. An example is illustrated below.
X={1,2,3,4,5,6} for i,value in enumerate(X): print(i,value)
Output: 0,1 1,2 2,3 3,4 4,5 5,6
Iterating a tuple using for loop
Iterating a tuple is like the list iteration using for loop. An example is illustrated below.
X=(1,2,3,4,5,6) for i,value in enumerate(X): print(i,value)
Output: 0,1 1,2 2,3 3,4 4,5 5,6
Iterating a dictionary using for loop
Iterating a dictionary is different from the other data types, as the dictionary contains key-value pairs. Hence to get just keys we use dictionaryname.keys() and for values we use dictionaryname.values(). An example is illustrated below.
X={“1”:1,”2”:2} for key in X.keys(): print(key) for value in X.values(): print(value) for key,value in X.items(): print(key,value)
Output: 1 2 1 2 1,1 2,2
Nested loops in Python
Nested loops are useful when building a brute force solution to a given problem. They increase time complexity of the program and decrease readability.
a = [1, 2] b = [10, 13] # getting numbers whose product is 13 for i in a: for j in b: if i*j == 13: print(i, j)
In above coding block, we defined 2 lists and each list has some collection of numbers. The main aim was to find what numbers product will be 13 from both the lists and also to print those numbers. For this purpose, we have to iterate through 2 lists, hence 2 for loops were used.
Alternative way:
There is a function in itertools which is called product. This helps in keeping the nested for loops if present in the program readable. The example is illustrated below.
from itertools import product
a = [1, 2]
b = [10, 13]
# getting numbers whose product is 13
for i, j in product(a, b):
if(i*j == 13): print(i, j)
While Loops in Python
Up till now, we have just printed out the output but never gave any input to our program. In Python input() is used for giving input to the program in ython. The exam is illustrated below. The while loop is used when you want to execute a program if the condition is fulfilled. While loop examples are illustrated below.
Printing 0-9 using while loop:
i = 0
while(i < 10): print(i) i += 1
As you can see the syntax is while followed by a condition, and inside the loop we increment the iterator according to the desired number.
Output: 0 1 2 3 4 5 6 7 8 9
In this blog, we have covered some basics of looping statements in python, the further topics on functions will be covered in next blog. The question to be solved is given below.
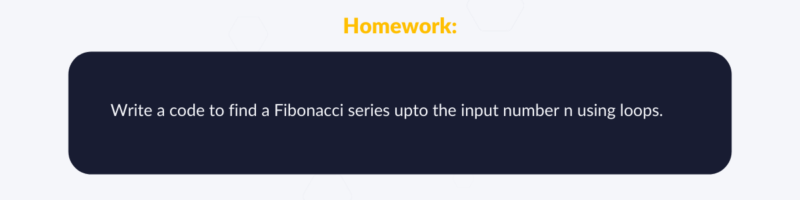
You may also like our JavaScript Course from Beginner to Advanced.
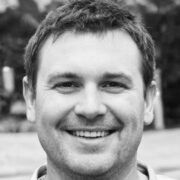
Author: Robert Whitney
JavaScript expert and instructor who coaches IT departments. His main goal is to up-level team productivity by teaching others how to effectively cooperate while coding.
Python Course From Beginner to Advanced in 11 blog posts:
- Introduction to Python Course. Part 1 Python Course from Beginner to Advanced in 11 blog posts
- Variables and Data Types in Python. Part 2 Python Course from Beginner to Advanced in 11 blog posts
- Python tuples, lists, sets and dictionaries. Part 3 Python Course from Beginner to Advanced in 11 blog posts
- Python sets and dictionaries. Part 4 Python Course from Beginner to Advanced in 11 blog posts
- Conditional statements in Python. Part 5 Python Course from Beginner to Advanced in 11 blog posts
- Loops in Python. Part 6 Python Course from Beginner to Advanced in 11 blog posts
- Python functions. Part 7 Python Course from Beginner to Advanced in 11 blog posts
- Advanced functions in Python. Part 8 Python Course from Beginner to Advanced in 11 blog posts
- Python classes and objects. Part 9 Python Course from Beginner to Advanced in 11 blog posts
- Files in Python. Part 10 Python Course from Beginner to Advanced in 11 blog posts
- Python applications in practice. Part 11 Python Course from Beginner to Advanced in 11 blog posts