This article will help the reader understand the basic Python classes along with some basic applications in real world. We will be using Visual Studio Code as our code editor. If you have not installed Visual Studio Code, the instructions are given in the first blog.
Python classes and objects – table of contents:
- Python classes
- Python classes – definition
- Initialization of Python classes
- Let’s write our first Python class
- Attributes
- Behavior of the class
- Objects in Python
- Inheritance
Python classes
As we have discussed in the first blog, Python is an object-oriented programming language. There are three phrases that are very important while discussing object-oriented programming in Python. The first one is class, the second one would is an object, the third one would be the inheritance. Let’s start with what is a class.
Python classes – definition
A class is a blueprint or an extensible program that helps in the creation of objects. A class consists of behavior and state. The behavior of a class is demonstrated through functions inside the class which are called methods. The state of the class is demonstrated using the variables inside the class which are called attributes.
Initialization of Python classes
A class can be initialized using the following syntax.
A class in python is defined using “class” keyword following the class name. The basic syntax of Python function is illustrated below.
For Example:
<img src="https://firmbee.com/wp-content/uploads/Python_9-800x600.png" alt="Python_classes" width="800" height="600" class="alignnone size-medium wp-image-21409 img-fluid" /> # Create a function # class Monkey class classname:
Note: class name is also having the same norms as the variable declaration.
Let’s write our first Python class
# first class class Animals: pass
In the above code block, we have written a class which we will be developing further in the blog. As you can see, we have used “class” keyword.
Let’s now see, how to add components to the animal’s class. But before that let’s learn about the “__init__()” constructor. Constructors are used for object instantiation. Here the __init__() is used for object instantiation. The constructor can be default with only self as an argument or parametrized with required arguments.
Attributes
There are two different types of attributes, the first ones are class variables and the second ones are instance variables. The class variables are the variables that are owned by the class. Also, these variables are available to all instances of the class. Hence, their value will not change even if the instance changes.
# class variables class Animals: type=”mammals”
The instance variables are the variables that belong to the instances itself. Hence, they will change their value as the instance changes.
# class variables class Animals: def __init__(self,legs): self.legs=legs
Note:Instance variables are not available for access using class name, because they change depending on the object accessing it.
Let’s make a program that has both class and instance variables declared.
class Animals: type=”mammals” def __init__(self,name,legs): self.name=name self.legs=legs
In the above program, we have used both instance and class variables. So, these variables form attributes of the class.
Behavior of the class
As discussed, behavior of the class is defined by the methods inside the class. But before going into the discussion on behavior, we have to start discussing the “self” parameter, which we used in the __init__().
Self:
In a very simple term, whenever we attach anything to self it says that the variable or function belongs to that class. Also, with “self”, the attributes or methods of the class can access.
Methods:
Class methods are functions inside the class which will have their first argument as “self”. A method inside the class is defined using “def” keyword.
class Animals: type=”mammals” def __init__(self,name,legs): self.name=name self.legs=legs def bark(self): if self.name==”dog”: print(“woof woof!!!”) else: print(“not a dog”)
In the above method “bark”, as we are using the name variable, which is an instance variable, we are accessing it using “self” and this function would print “woof woof!!!”, only if the name provided to the object, is dog.
We have discussed most of the components of a class, but you might be thinking how to see if the class is working. The answer to this is unless we create an object of the class, we will not be able to see what the class is doing. Now, Let’s define and create an object of the class.
Objects in Python
An object is an instance of the class. A class is just a blueprint, but the object is an instance of the class which has actual values.
The code for defining or creating an object is illustrated below.
class Animals: type=”mammals” def __init__(self,name,legs): self.name=name self.legs=legs def bark(self): if self.name==”dog”: print(“woof woof!!!”) else: print(“not a dog”) dog=Animals(“dog”,4)
To create an object, the syntax is the objectname=classname(arguments). Hence, here we are giving the name of the animal to be dog and number of legs to be 4. Now, the object of the class is created, the next step is to use the object to access its attributes. To access the attributes of a class using the object, remember only the instance variables can be accessed using the object. The instance variables in our class are name and legs.
class Animals: type=”mammals” def __init__(self,name,legs): self.name=name self.legs=legs def bark(self): if self.name==”dog”: print(“woof woof!!!”) else: print(“not a dog”) dog=Animals(“dog”,4) print(dog.name) print(dog.legs)
As we can see, we are able to access instance variables using dot notation.
Let’s explore the output.
#Output dog 4
To access the functions inside the class or methods, we will be using the dot notation. The example is illustrated below.
class Animals: type=”mammals” def __init__(self,name,legs): self.name=name self.legs=legs def bark(self): if self.name==”dog”: print(“woof woof!!!”) else: print(“not a dog”) dog=Animals(“dog”,4) print(dog.name) print(dog.legs) print(dog.bark())
#Output dog 4 woof woof!!!
In the above example, we can see that we are accessing the class method “bark” using the dog object we created. We can see that we are not using the “self” parameter in the function arguments. This is because we don’t require the use of “self” outside the class as the object itself is acting as self.
Inheritance
Inheritance is a process through which the class attributes and methods can be passed to a child class. The class from where the child class is inheriting is the parent class. The syntax for inheritance is illustrated below.
#Inheritance class parent: class child(parent):
From the above illustration, we can see that for the inheritance syntax we are placing the parent class name as an argument to the child class. Let’s use the Animals class and make a child class called dog. This is illustrated below.
class Animals: type=”mammals” def __init__(self,name,legs): self.name=name self.legs=legs def bark(self): if self.name==”dog”: print(“woof woof!!!”) else: print(“not a dog”) class Dog(Animals): def __init__(self,name,legs,breed): Animals.__init__(self,name,legs) self.breed=breed
In the above example code, we made a dog class which is extending the animals class which we created before. We are also using the parameters from the Animals using the Animals.__init__(arguments) which has name and legs which will be inherited to the dog class. Then we are making an instance attribute for the dog class which is breed.
Now let’s make an object for the dog class and access the attributes and methods of the animals class.
class Animals: type=”mammals” def __init__(self,name,legs): self.name=name self.legs=legs def bark(self): if self.name==”dog”: print(“woof woof!!!”) else: print(“not a dog”) class Dog(Animals): def __init__(self,name,legs,breed): Animals.__init__(self,name,legs) self.breed=breed pug=Dog("dog",4,"pug") pug.breed pug.name pug.legs pug.bark()
#Output pug dog 4 woof woof!!!
As we can see from the output the parent class attributes and methods are being accessed by the child class object.
In this blog, we have covered some basics of classes in Python. In the next blog post we will cover the topic of file handling.
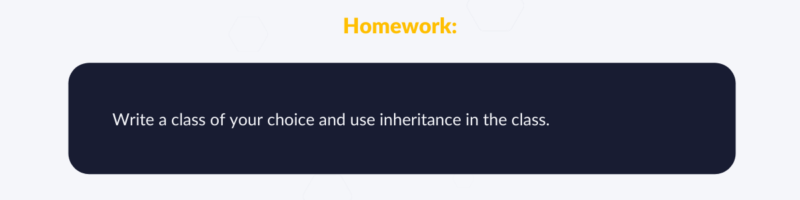
You may also like our JavaScript Course from Beginner to Advanced.
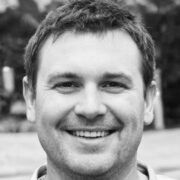
Author: Robert Whitney
JavaScript expert and instructor who coaches IT departments. His main goal is to up-level team productivity by teaching others how to effectively cooperate while coding.
Python Course From Beginner to Advanced in 11 blog posts:
- Introduction to Python Course. Part 1 Python Course from Beginner to Advanced in 11 blog posts
- Variables and Data Types in Python. Part 2 Python Course from Beginner to Advanced in 11 blog posts
- Python tuples, lists, sets and dictionaries. Part 3 Python Course from Beginner to Advanced in 11 blog posts
- Python sets and dictionaries. Part 4 Python Course from Beginner to Advanced in 11 blog posts
- Conditional statements in Python. Part 5 Python Course from Beginner to Advanced in 11 blog posts
- Loops in Python. Part 6 Python Course from Beginner to Advanced in 11 blog posts
- Python functions. Part 7 Python Course from Beginner to Advanced in 11 blog posts
- Advanced functions in Python. Part 8 Python Course from Beginner to Advanced in 11 blog posts
- Python classes and objects. Part 9 Python Course from Beginner to Advanced in 11 blog posts
- Files in Python. Part 10 Python Course from Beginner to Advanced in 11 blog posts
- Python applications in practice. Part 11 Python Course from Beginner to Advanced in 11 blog posts